Netmiko is multi-vendor networking Python library and is based on Paramiko which is the standard Python SSH library. Netmiko supports multiple vendors, one of them being Cisco (IOS, NX-OS, ASA, IOS-XE, IOS-XR), Juniper Junos, Linux, Palo Alto PanOS, and others. Netmiko is very useful to execute show commands to multiple devices and also to configure them via SSH or even Telnet. In this post we show the steps to configure Cisco routers to accept SSH connections, install necessary packages on a Linux instance already created in GNS3, and run a simple script that uses Netmiko to connect to a Cisco Router in GNS3 and execute a show command.
1.- Configure the router or routers so that they accept ssh connections:
line vty 0 4 login local transport input ssh ip domain name cisco.com username cisco password cisco enable secret cisco ip ssh rsa keypair-name cisco crypto key generate rsa usage-keys label cisco modulus 1024 ip ssh version 2
2.- Configure the linux instance so that it uses ssh v2 by opening /etc/ssh/ssh_config in a text editor and uncommenting “Protocol 2” line:
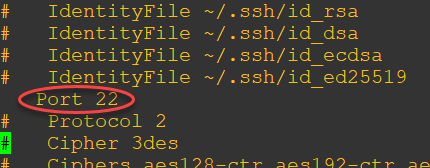
3.- Upgrade necessary packages to install python 3.7 and netmiko. In my testings netmiko only worked with python 3.6 and above.
apt-get update
apt-get install software-properties-common
apt-get install sudo
sudo add-apt-repository ppa:deadsnakes/ppa
sudo apt-get update
sudo apt-get install python3.7
sudo apt-get install python3-pip
sudo update-alternatives --install /usr/bin/python3 python3 /usr/bin/python3.7
pip3 install --upgrade pip
pip3 install netmiko
4.- Time to test it. Open a vi or nano editor and write the following script and save it as test.py:
#!/usr/bin/env python
from netmiko import Netmiko
from netmiko import ConnectHandler
from getpass import getpass
cisco_router = {
'device_type': 'cisco_ios',
'host': '10.0.0.1',
'username': 'cisco',
'password': getpass(),
'port' : 22, # optional, defaults to 22
'secret': 'cisco', # optional, defaults to ''
}
net_connect = ConnectHandler(**cisco_router)
output = net_connect.send_command('show ip int brief')
print(output)
5.- Run the script:
root@UbuntuDockerGuest-2:~# python3.7 test.py
Password:
Interface IP-Address OK? Method Status Protocol
GigabitEthernet0/0 172.18.123.2 YES NVRAM up up
GigabitEthernet0/1 172.18.23.5 YES NVRAM up up
GigabitEthernet0/2 unassigned YES NVRAM up up
GigabitEthernet0/2.50 10.50.1.12 YES NVRAM up up
GigabitEthernet0/2.199 172.18.215.9 YES NVRAM up up
GigabitEthernet0/3 10.40.1.139 YES DHCP up up
NVI0 172.18.123.2 YES unset up up
This is just a simple script. In future posts we are going to look at scripts that get show command outputs from multiple devices.
References
https://pypi.org/project/netmiko/